Binary search time complexity is one of the reasons why binary search is much faster than linear search, especially when dealing with larger datasets. While linear search checks each element one by one, binary search divides the dataset in half with each step. This makes it a more efficient choice for sorted data. In this blog, we will break down the binary search time complexity and explain why it’s considered one of the fastest search algorithms.
The time complexity of binary search is O(log n), which means it gets faster as the size of the data increases. This is much better than linear search, which has a time complexity of O(n). In simple terms, binary search reduces the number of steps it takes to find an element by cutting the search space in half each time. Let’s explore what this really means and how you can apply binary search to improve your code’s performance.
What is Binary Search Time Complexity
Binary search time complexity refers to how quickly binary search can find an element in a sorted list. The main reason binary search is faster than linear search is because it doesn’t look at every item. Instead, it divides the list into smaller parts, and checks the middle item each time. This makes it a very fast way to find something in large lists.
When we talk about time complexity, we use terms like O(log n) to describe how the algorithm’s performance changes as the list grows. In simple words, O(log n) means that with each step, the amount of work gets cut in half. So, the bigger the list, the fewer steps it takes to find the item.
The time complexity of binary search is one of the reasons why it is a favorite among programmers when they need to search through large datasets
How Does Binary Search Time Complexity Compare to Linear Search
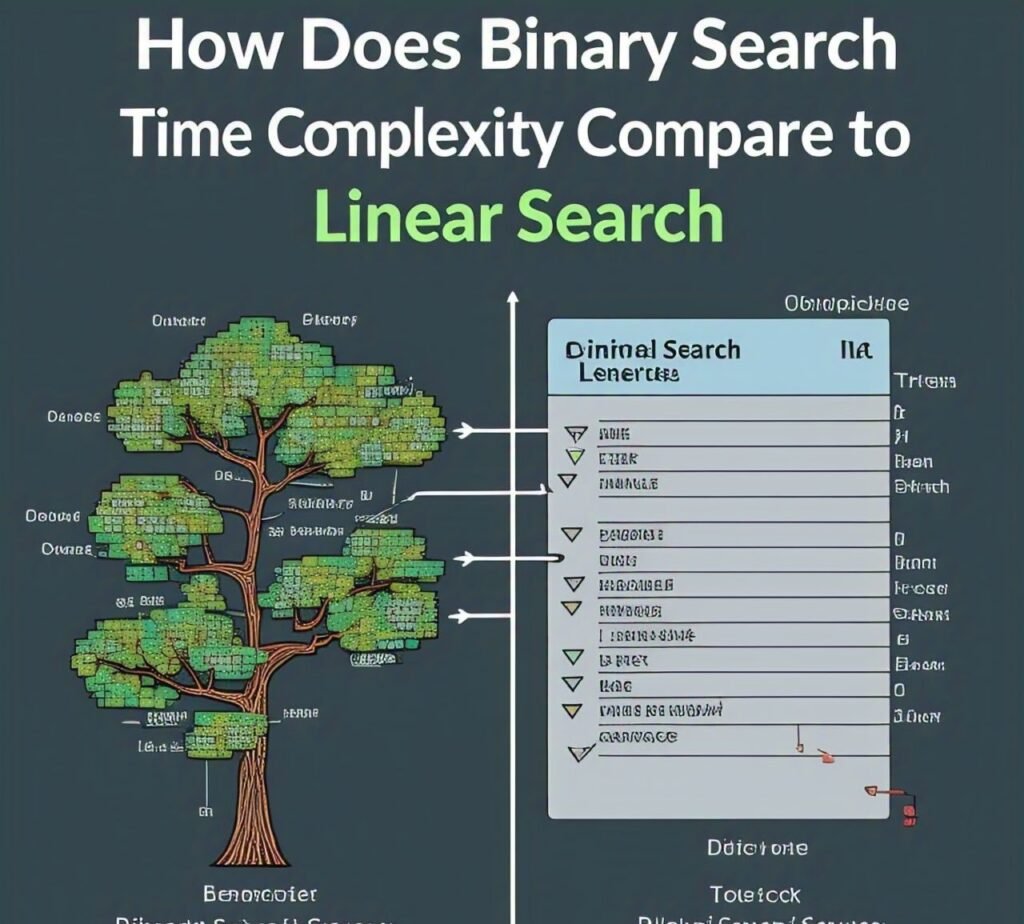
The difference between binary search and linear search comes down to how they handle data. Linear search is simple; it goes through each element, one by one, until it finds the right one. This means that its time complexity is O(n), which gets slower as the list grows larger.
On the other hand, binary search reduces the problem size with every step. So, even though the list may have thousands of items, binary search will still find the item much faster. The time complexity of binary search is O(log n), which means the number of steps grows much slower as the size of the list increases.
Why Binary Search Time Complexity Makes It Ideal for Large Datasets
Binary search time complexity makes it perfect for working with large datasets. When you have a list with thousands or even millions of items, searching through each item one by one would take a long time. But binary search makes it much faster by dividing the list into smaller and smaller parts.
For example, imagine you are looking for a book in a library with thousands of books. Instead of checking each shelf one by one, you start by checking the middle shelf. From there, you keep cutting the number of shelves in half. This means fewer steps are needed to find the book you’re looking for.
Reduces steps needed to find an item.
Works well for large data sets.
Faster than linear search for bigger lists.
Understanding the O(log n) in Binary Search Time Complexity
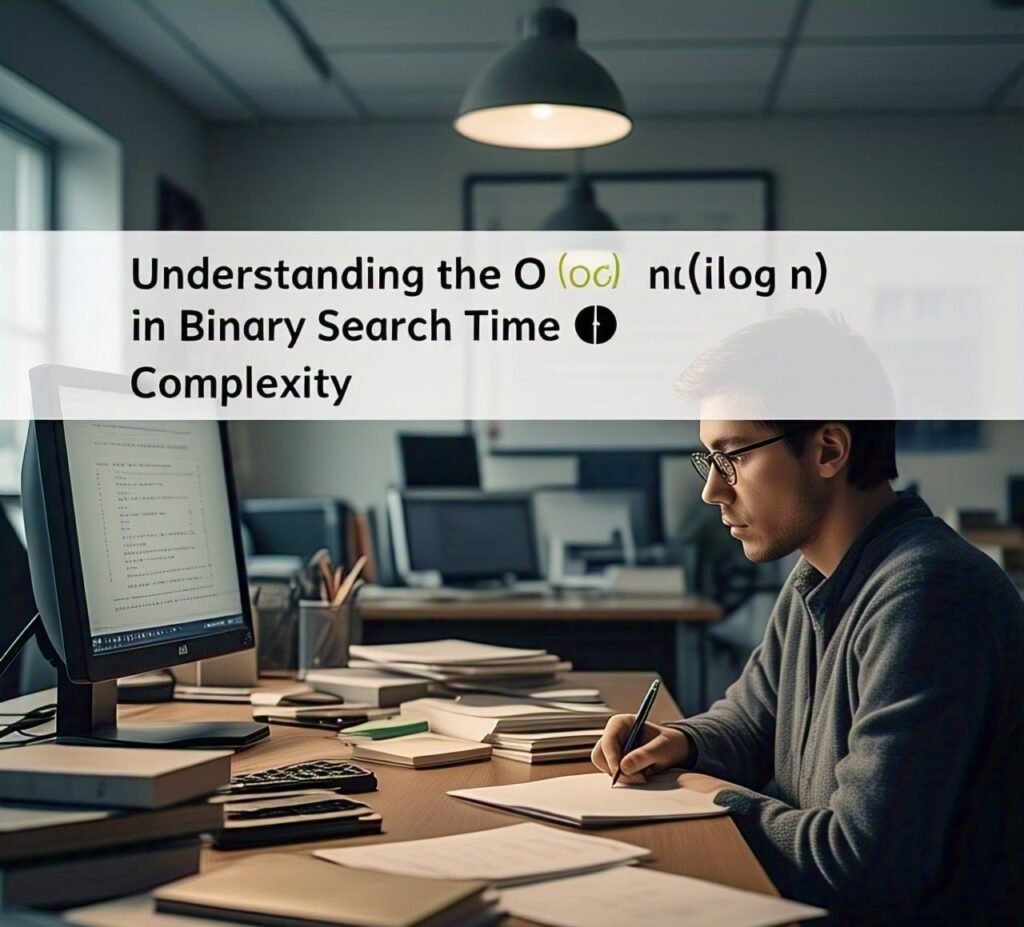
When we say that binary search time complexity is O(log n), we’re talking about how the algorithm’s steps grow as the size of the list increases. The “log” part refers to the fact that with every step, binary search cuts the problem in half. For example, if there are 100 items, binary search might only need 7 steps to find the right one, because the number of possible choices gets halved each time.
The “n” in O(log n) refers to the number of items in the list. But don’t worry—logarithms can be a bit tricky! The key thing to remember is that as the list gets bigger, the number of steps binary search needs grows very slowly. This makes it much faster than linear search, especially for large datasets
Conclusion
In conclusion, binary search time complexity makes it one of the fastest ways to search through large datasets. By dividing the dataset in half with every step, binary search can find an element in much fewer steps than linear search, especially when the dataset is large. This makes it perfect for applications where speed is important.
Remember, binary search only works if the data is sorted. Once the list is sorted, binary search will help you search efficiently with its O(log n) time complexity. It’s a powerful tool for developers, especially when dealing with large datasets.
FAQs
Q: What is binary search time complexity
A: Binary search time complexity is O(log n), which means it reduces the search space by half with each step, making it fast for large datasets.
Q: How does binary search differ from linear search
A: Binary search is faster than linear search because it divides the dataset in half with each step, whereas linear search checks every item one by one.
Q: Can binary search work on unsorted data
A: No, binary search only works on sorted data. If the data is unsorted, you must sort it first.
Q: Why is binary search faster than linear search
A: Binary search is faster because it reduces the number of comparisons needed by halving the dataset at each step, while linear search checks each item individually.
Q: When should I use binary search
A: Use binary search when you have a sorted list and need to find an item quickly. It is ideal for large datasets.